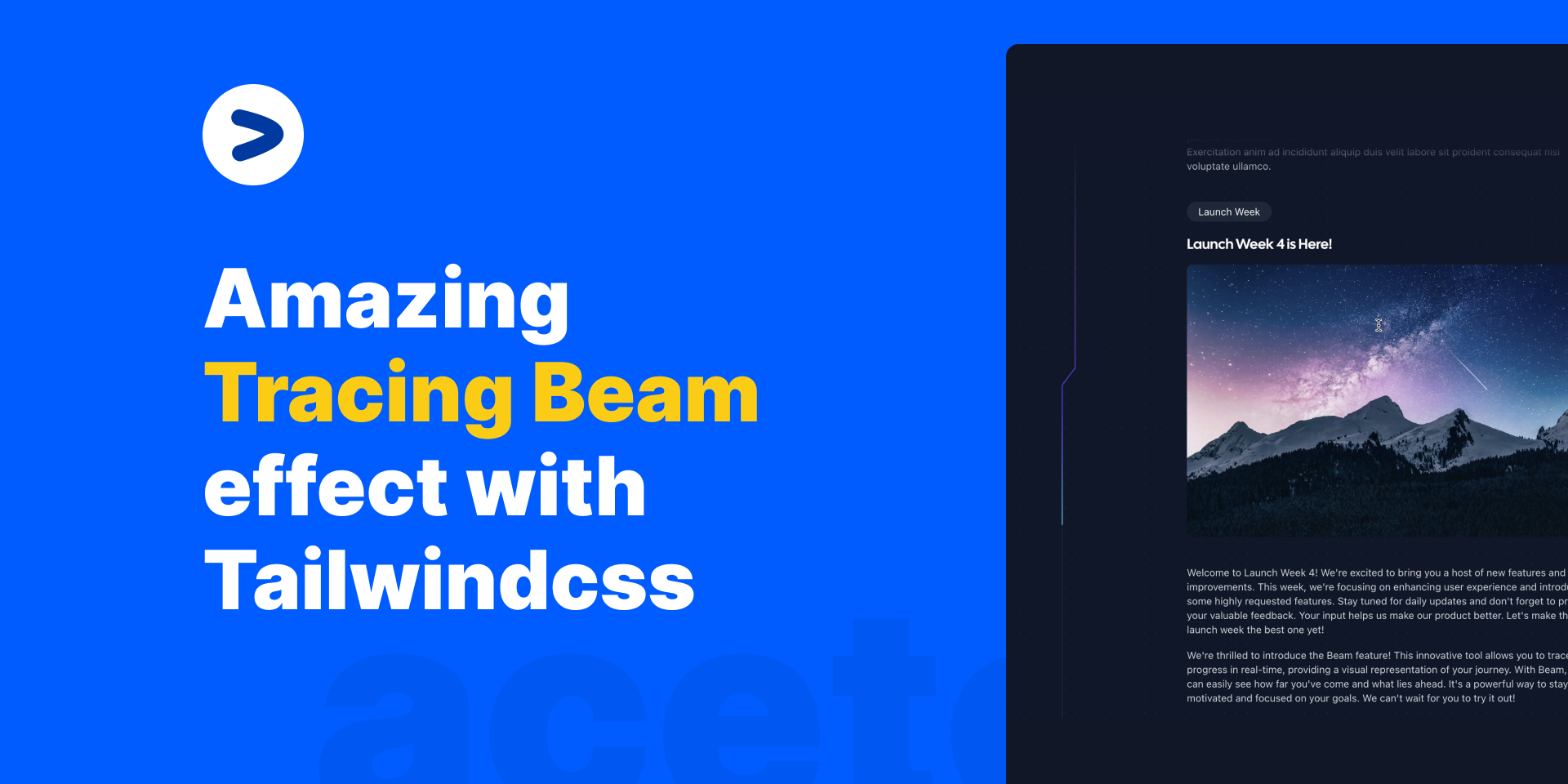
Amazing Tracing Beam Effect With Tailwindcss
Recently, I came across Clerk's Changelog website. There, I noticed a small gradient beam that was following the path of an SVG. It immediately caught my attention because of how unique it was and it was following the user's scroll.
I tried to recreate it using Tailwindcss and Framer Motion.
Here's a breakdown of how I did it 👇
Preview
React
Lorem Ipsum Dolor Sit Amet
Sit duis est minim proident non nisi velit non consectetur. Esse adipisicing laboris consectetur enim ipsum reprehenderit eu deserunt Lorem ut aliqua anim do. Duis cupidatat qui irure cupidatat incididunt incididunt enim magna id est qui sunt fugiat. Laboris do duis pariatur fugiat Lorem aute sit ullamco. Qui deserunt non reprehenderit dolore nisi velit exercitation Lorem qui do enim culpa. Aliqua eiusmod in occaecat reprehenderit laborum nostrud fugiat voluptate do Lorem culpa officia sint labore. Tempor consectetur excepteur ut fugiat veniam commodo et labore dolore commodo pariatur.
Dolor minim irure ut Lorem proident. Ipsum do pariatur est ad ad veniam in commodo id reprehenderit adipisicing. Proident duis exercitation ad quis ex cupidatat cupidatat occaecat adipisicing.
Tempor quis dolor veniam quis dolor. Sit reprehenderit eiusmod reprehenderit deserunt amet laborum consequat adipisicing officia qui irure id sint adipisicing. Adipisicing fugiat aliqua nulla nostrud. Amet culpa officia aliquip deserunt veniam deserunt officia adipisicing aliquip proident officia sunt.
Aute ut laborum sint consequat velit excepteur sunt ex aliquip est consectetur. In adipisicing ea in consectetur qui dolore dolor sint aliquip esse. Laboris excepteur velit reprehenderit do nulla ipsum magna in esse sit cillum excepteur anim anim. Ea dolore labore officia sit enim consequat nisi occaecat nisi tempor. Culpa minim cillum incididunt culpa nisi ipsum non laborum tempor ad. Est aute id ipsum aute et et.
Sit cupidatat incididunt aute ad commodo culpa ad aliqua voluptate dolor. Minim ut anim nostrud voluptate do officia enim incididunt. Deserunt consequat veniam cillum ipsum commodo anim.
Ut aliquip ipsum duis Lorem labore do non ullamco laborum amet fugiat in pariatur. Ut ut voluptate esse esse mollit ullamco dolor aliquip eiusmod nostrud ullamco enim duis. Laborum excepteur sint occaecat sint laboris ut laborum velit ut irure aliqua. Ad velit reprehenderit et cillum dolore duis duis quis. Eiusmod consectetur ad proident velit commodo cupidatat. Cupidatat quis id anim in nulla. Esse ullamco consequat nisi ipsum quis pariatur ipsum culpa labore id sint.
Aliquip esse elit aliqua culpa aliquip labore culpa. Aute aliquip id ex consequat in irure laboris consectetur esse. Adipisicing elit occaecat cillum elit dolor eiusmod pariatur Lorem id sint tempor consectetur. Excepteur magna ipsum dolor deserunt aliqua aliquip irure. Elit ut consectetur officia ut cupidatat sit reprehenderit pariatur consequat. Voluptate elit est nostrud eu anim excepteur officia. Incididunt cupidatat tempor ut sunt occaecat amet labore consectetur mollit.
Changelog
Lorem Ipsum Dolor Sit Amet
Ex irure dolore veniam ex velit non aute nisi labore ipsum occaecat deserunt cupidatat aute. Enim cillum dolor et nulla sunt exercitation non voluptate qui aliquip esse tempor. Ullamco ut sunt consectetur sint qui qui do do qui do. Labore laborum culpa magna reprehenderit ea velit id esse adipisicing deserunt amet dolore. Ipsum occaecat veniam commodo proident aliqua id ad deserunt dolor aliquip duis veniam sunt.
In dolore veniam excepteur eu est et sunt velit. Ipsum sint esse veniam fugiat esse qui sint ad sunt reprehenderit do qui proident reprehenderit. Laborum exercitation aliqua reprehenderit ea sint cillum ut mollit.
Tempor velit ullamco sit proident ut. Consectetur commodo eiusmod sit culpa occaecat pariatur velit minim. Nulla minim et ea qui tempor dolore et pariatur nulla aute nulla. Exercitation anim ad incididunt aliquip duis velit labore sit proident consequat nisi voluptate ullamco.
Launch Week
Lorem Ipsum Dolor Sit Amet
Ex irure dolore veniam ex velit non aute nisi labore ipsum occaecat deserunt cupidatat aute. Enim cillum dolor et nulla sunt exercitation non voluptate qui aliquip esse tempor. Ullamco ut sunt consectetur sint qui qui do do qui do. Labore laborum culpa magna reprehenderit ea velit id esse adipisicing deserunt amet dolore. Ipsum occaecat veniam commodo proident aliqua id ad deserunt dolor aliquip duis veniam sunt.
In dolore veniam excepteur eu est et sunt velit. Ipsum sint esse veniam fugiat esse qui sint ad sunt reprehenderit do qui proident reprehenderit. Laborum exercitation aliqua reprehenderit ea sint cillum ut mollit.
Tempor velit ullamco sit proident ut. Consectetur commodo eiusmod sit culpa occaecat pariatur velit minim. Nulla minim et ea qui tempor dolore et pariatur nulla aute nulla. Exercitation anim ad incididunt aliquip duis velit labore sit proident consequat nisi voluptate ullamco.
As you can see, the beam is following the path of the SVG. But it's not really the case. The beam is not "following" anything per se.
It is a linear gradient
that is applied on the svg. The gradient moves with user scroll. We animate the y1
and y2
values of the gradient
and that is the reason it appears to be moving on scroll.
Structure
This is the basic code structure of the page. Here, we have a Content
section which is nothing but a blog. It can be any piece of content or section
that you want to have at the right side of the page.
We have the svgHeight
state variable. This is to set the height of the SVG. We need to set the height of the SVG because we want the gradient to cover the entire height of the content section. Also, We want to basically curve
the svg at the bottom of the section
and hence, need to figure out the height of the svg.
To find the height of the SVG, we use ref
to measure the height of the content section. We use useRef
to create a ref for the content section and then use useEffect
to measure the height of the content section when the component mounts.
The code we are interested in is this 👇
This is the linear gradient that we are going to animate.
Animating using framer motion
We follow a 3 step process to animate the gradient.
- We use
useScroll
hook to get the scroll progress of the container. This will be a value between 0 and 1. As and when the user scroll through the page, the value changes from 0 to 1. - We use
useTransform
hook to transform the scroll progress value to a value between 50 and the height of the SVG. This will be the value ofy1
andy2
of the gradient. For example, the code snippet
const y1 = useSpring(
useTransform(scrollYProgress, [0, 1], [50, svgHeight - velo * 500]),
{
stiffness: 500,
damping: 90,
// duration: 3,
}
);
will transform the scroll progress value to a value between 50 and svgHeight - velo * 500
. The velo
variable is the velocity of the scroll progress. We use this to make the gradient move faster or slower depending on the scroll velocity.
useSpring
is another hook which makes the animation smooth. We pass the transformed value to the y1
and y2
of the gradient.
- Once we have the
y1
andy2
values, we pass it to thegradient
element.
<defs>
<motion.linearGradient
id="gradient"
gradientUnits="userSpaceOnUse"
x1="0"
x2="0"
y1={y1}
y2={y2}
transition={{
duration: 10,
}}
>
<stop stopColor="#18CCFC" stopOpacity="0"></stop>
<stop stopColor="#18CCFC"></stop>
<stop offset="0.325" stopColor="#6344F5"></stop>
<stop offset="1" stopColor="#AE48FF" stopOpacity="0"></stop>
</motion.linearGradient>
</defs>
Result
The result is a beam that follows the path (sort of 😉).
Please note that we are using container
and offset
in useScroll
hook's options. This is because we have an overflowing container in the preview.
If you want to track scroll of the entire page, simply remove the options
from the useScroll hook and it will work as intended for the entire page. The code will look like this:
const { scrollYProgress } = useScroll();
Conclusion
If you want the complete code snippet with a working demo, checkout the Tracing Beam Effect With Tailwindcss and Framer Motion on aceternity, I've written complete code with preview for this effect.
This was a fun little experiment. I hope you enjoyed it. If you have any questions, feel free to reach out to me on Twitter.

posted by Manu Arora