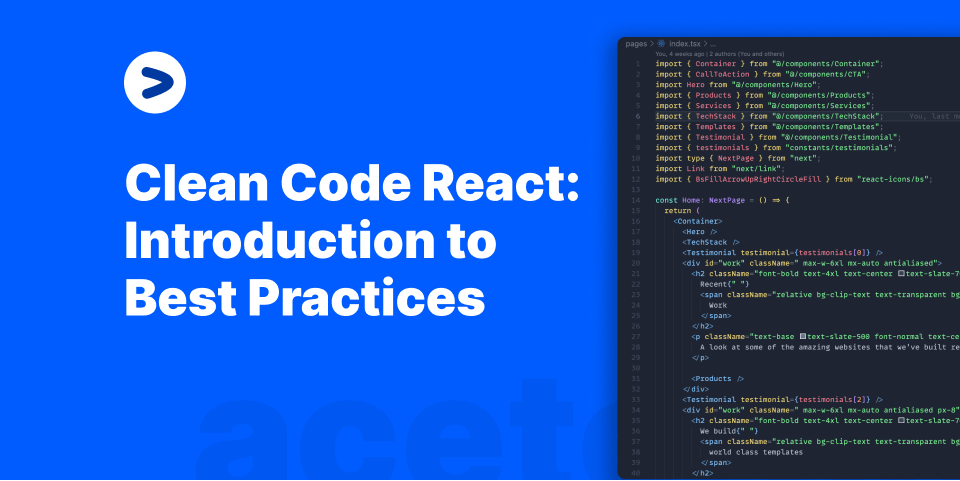
Clean Code React: Introduction to Best Practices
I. Introduction
React is a popular JavaScript library for building user interfaces, particularly in single-page applications. As React projects grow larger and more complex, it becomes increasingly important to write clean and maintainable code. Clean code makes it easier for other developers to understand, modify, and extend your codebase, ultimately leading to better collaboration and a more efficient development process.
In this blog post, we will discuss best practices for writing clean code in React, including component organization, state management, type checking, and styling. We will also provide tips and recommendations for each topic, so you can start improving your React code today.
II. Components
Components are the building blocks of a React application, and organizing them effectively is crucial for clean code. Let's dive into functional vs. class components, component structure and organization, and naming conventions.
A. Functional components vs. class components
There are two types of components in React: functional components and class components. Functional components are plain JavaScript functions that return JSX, while class components are ES6 classes that extend React.Component
.
1. Advantages of functional components
Functional components are generally preferred over class components for several reasons:
- They are more concise and easier to read.
- They encourage the use of immutable data through props.
- They allow for better separation of concerns by encouraging the use of hooks for managing state and side effects.
2. When to use class components
Class components should only be used when it's necessary to use React's lifecycle methods or when working with legacy code that requires the use of class components.
B. Component structure and organization
Organizing your components effectively is essential for clean React code.
1. Single responsibility principle
Following the single responsibility principle means that each component should have one primary responsibility or purpose. This makes it easier to understand what a component does, and it helps to keep your codebase modular and maintainable.
2. Component composition
Component composition refers to the practice of building complex components by combining smaller, more focused components. This approach allows for better code reuse and separation of concerns. When creating new components, always look for opportunities to break them down into smaller, more focused subcomponents.
C. Naming conventions
Having consistent and descriptive naming conventions for your components is crucial for readability and maintainability. Some general guidelines include:
- Use PascalCase for component names (e.g.,
MyComponent
). - Be descriptive and avoid abbreviations.
- Keep component names short and concise, but avoid single-letter names.
III. State Management
Managing state effectively is critical for clean React code. Let's discuss useState and useEffect hooks, useContext and useReducer hooks, and third-party state management libraries.
A. useState and useEffect hooks
React hooks have revolutionized the way we manage state and side effects in functional components.
1. Benefits of using hooks
Hooks have several advantages over traditional class-based state management:
- They allow for better separation of concerns by isolating stateful logic within individual hooks.
- They enable the use of state and side effects in functional components, eliminating the need for class components in most cases.
- They make it easier to share stateful logic between components.
2. Tips for using hooks effectively
- Use the
useState
hook for local component state. - Use the
useEffect
hook for side effects such as fetching data or updating the DOM. - Avoid using too many hooks in a single component; instead, consider creating custom hooks to encapsulate related stateful logic.
B. useContext and useReducer hooks
In more complex applications, you may need to manage state that is shared across multiple components or requires more sophisticated state management logic.
1. When to use context and reducers
Consider using the useContext
and useReducer
hooks when:
- You need to share state across multiple components in a component tree.
- Your state logic is complex and requires more than simple
useState
updates.
2. Best practices for managing complex state
- Use the
useContext
hook to provide access to your global state. - Use the
useReducer
hook to manage complex state transitions and side effects. - Keep your reducers pure and free of side effects.
C. Third-party state management libraries
Several third-party libraries can help you manage state in your React applications, including Redux and MobX.
1. Redux
Redux is a popular state management library that uses a single, immutable store to manage application state. It is particularly well-suited for large applications with complex state management requirements.
2. MobX
MobX is another state management library that uses observables to manage state changes in a more reactive way. It is often considered easier to learn and use than Redux, especially for smaller applications.
3. Criteria for choosing a state management library
Consider the following factors when choosing a state management library:
- The size and complexity of your application
- Your team's familiarity with the library
- The library's community, documentation, and ecosystem
IV. PropTypes and Type Checking
Using PropTypes or TypeScript can help catch bugs early and improve the maintainability of your React code.
A. Importance of type checking
Type checking ensures that the data being passed between components is of the correct type, making it easier to catch bugs before they cause issues in your application.
B. Using PropTypes
PropTypes is a built-in package for type checking in React.
1. Defining PropTypes for components
Define PropTypes for each component to ensure that the correct data types are being passed as props:
import PropTypes from 'prop-types';
MyComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number,
2. Tips for using PropTypes effectively
- Always define PropTypes for your components, even if they are simple.
- Use
isRequired
to enforce the presence of required props. - Use custom validators to handle more complex validation requirements.
C. TypeScript with React
TypeScript is a popular superset of JavaScript that adds static type checking.
1. Benefits of using TypeScript
Using TypeScript with React provides several benefits:
- Improved code quality with compile-time type checking.
- Better tooling and editor support for autocompletion and error checking.
- Easier refactoring and code navigation.
2. Integrating TypeScript in a React project
To integrate TypeScript in a React project:
- Create a new React project with TypeScript using
create-react-app my-app --template typescript
. - Install the necessary TypeScript dependencies.
- Configure your TypeScript settings in the
tsconfig.json
file.
V. Styling and CSS
Writing clean and maintainable CSS is just as important as writing clean React code. There are several approaches to styling React components, including CSS modules, styled-components, and emotion.
A. CSS Modules
CSS Modules allow you to write modular and scoped CSS that is automatically scoped to individual components. This helps prevent naming conflicts and makes it easier to manage styles across your application.
B. Styled-components and emotion
Styled-components and emotion are popular CSS-in-JS libraries that allow you to write scoped, dynamic styles directly in your components. They provide additional benefits such as theming support, server-side rendering, and improved developer experience.
C. Tips for clean CSS in React
- Choose a consistent styling approach for your project.
- Follow best practices for naming conventions, organization, and modularity.
- Use utility libraries like Tailwind CSS or Bootstrap for faster development and a consistent design system.
By following these best practices, you can write clean and maintainable React code that is easier to understand, modify, and extend. Embrace these principles to improve your development workflow and create better React applications.

posted by Manu Arora